【Java】リストからMAP変換!streamからのgroupingByでグループ化して値を保持するよ
今回はListからMapを作成してみます
TestDto型のリストを用意して、age(年齢)変数をキーにvalueへTestDtoリスト(21歳の従業員情報の集まり)を格納します
streamを使用し、一発で作成します!
// 従業員情報
public class TestDto {
private Integer id;
private String name;
private Integer age;
private Integer personId;
public TestDto(
Integer id,
String name,
Integer age,
Integer personId) {
this.id = id;
this.name = name;
this.age = age;
this.personId = personId;
}
}
⇑ まあこれはよくあるやつですね!!
ここからがロジックです
public static void outputPersonInfo(String[] args) {
// TestList型のリストを宣言
List<TestDto> testDtoList = new ArrayList<>();
// 従業員情報を作成(id, 名前+年齢, 年齢, 従業員番号)
TestDto testDto1 = new TestDto(1,"赤坂20", 20, 10001);
TestDto testDto2 = new TestDto(2,"井上21", 21, 10002);
TestDto testDto3 = new TestDto(3,"上原21", 21, 10003);
TestDto testDto4 = new TestDto(4,"江原20", 20, 10004);
TestDto testDto5 = new TestDto(5,"小野寺22", 22, 10005);
TestDto testDto6 = new TestDto(6,"加藤21", 21, 10006);
TestDto testDto7 = new TestDto(7,"木村20", 20, 10007);
TestDto testDto8 = new TestDto(8,"工藤22", 22, 10008);
// 従業員情報をリストにまとめます
testDtoList.add(testDto1);
testDtoList.add(testDto2);
testDtoList.add(testDto3);
testDtoList.add(testDto4);
testDtoList.add(testDto5);
testDtoList.add(testDto6);
testDtoList.add(testDto7);
testDtoList.add(testDto8);
// 作成したいMAPを宣言します(省略おっけですよ)
// Map<年齢, List<従業員情報>>
Map<Integer, List<TestDto>> personInfoMap = new HashMap<>();
// 従業員情報リストから年齢をキー(key)に従業員情報をまとめたMAPを1発で生成します
personInfoMap = testDtoList.stream().collect(Collectors.groupingBy(TestDto::getAge));
// personInfoMapから21歳の従業員情報を取得する
List<TestDto> personInfo21AgeList = personInfoMap.get(21);
// 21歳のユーザの「名前」を出力します
for(TestDto personInfo21Age : personInfo21AgeList) {
System.out.println(personInfo21Age.getName());
}
}
// 出力結果
// 井上21
// 上原21
// 加藤21
21歳の従業員情報を取得し「名前」を出力することができました!
このロジックで重要なのは以下のソースです
// 従業員情報リストから年齢をキー(key)に従業員情報をまとめたMAPを1発で生成します
personInfoMap = testDtoList.stream().collect(Collectors.groupingBy(TestDto::getAge));
従業員情報リスト(testDtoList)を「stream」で流し、「Collectors」の「.groupingBy」メソッドでキーを引数(TestDto::getAge)に入れると引数をキーとしたListのvalueを作成してくれるという代物です
Mapでグループ分けして、いろんなところで使用するというのはよくあるので、その時はぜひ試してみてください
ではっ
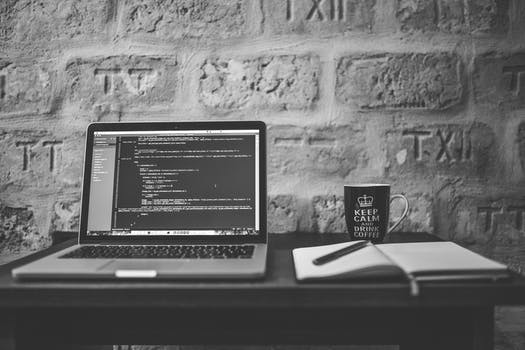
是非フォローしてください
最新の情報をお伝えします