【Python】curlコマンドを実行して外部システムにリクエストしたい
一般的にはrequestsライブラリを使うのがオススメです。
さまざまな例を紹介していきます。
1. requestsライブラリをインストール
インストールしていない場合は、以下を実行して「requests」をインストールします
pip install requests
2. シンプルなGETリクエストをします
「curl https://example.com」をGETで実行します。
import requests
response = requests.get('https://example.com')
# レスポンス内容を表示
print(response.status_code) # ステータスコード(例:200)
print(response.text) # レスポンスボディ(HTMLとかJSONとか)
3. POSTリクエストでデータ送信する場合
「curl -X POST -d “key=value” https://example.com」を実行します
import requests
payload = {'key': 'value'}
response = requests.post('https://example.com', data=payload)
print(response.status_code)
print(response.text)
4. 認証が必要な場合(例:ベーシック認証)
from requests.auth import HTTPBasicAuth
import requests
response = requests.get('https://example.com', auth=HTTPBasicAuth('username', 'password'))
print(response.status_code)
print(response.text)
5. ヘッダーをつけたい場合
「curl -H “Authorization: Bearer token” https://example.com」を実行します
import requests
headers = {
'Authorization': 'Bearer YOUR_TOKEN',
'Content-Type': 'application/json'
}
response = requests.get('https://example.com', headers=headers)
print(response.status_code)
print(response.text)
6. ハッシュ化してヘッダーに設定したい!
現在日時とある文字列を結合して、それをSHA-256に変換してヘッダーに設定して実行します。
import hashlib
import datetime
import requests
# 1. 現在日時を取得
now = datetime.datetime.utcnow().strftime('%Y%m%d%H%M%S') # UTC時刻で、フォーマット例: 20250428093045
# 2. 文字列を結合
fixed_string = "your_fixed_string"
target = now + fixed_string
# 3. SHA-256変換
hashed_value = hashlib.sha256(target.encode('utf-8')).hexdigest()
# 4. ヘッダーに設定してリクエスト
headers = {
'X-Custom-Hash': hashed_value, # ここはAPI仕様に合わせてヘッダー名を変える
'Content-Type': 'application/json'
}
response = requests.get('https://example.com', headers=headers)
# レスポンス確認
print(response.status_code)
print(response.text)
是非参考ください!
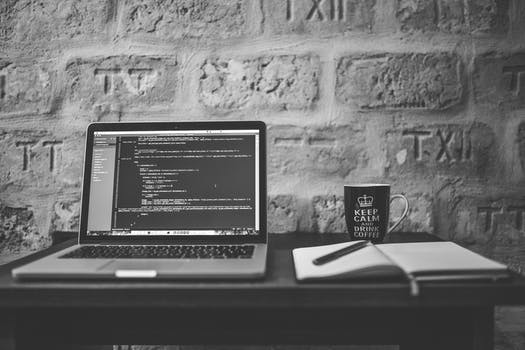
是非フォローしてください
最新の情報をお伝えします